TDA Access Policies - PowerShell Scripting options with examples
Learn how to use PowerShell scripting option to create powerful custom Access Policy rules
From TDA version 8.2.0, Access Policies allow you to utilise PowerShell to extract specific system information, and based on results, decide whether TDA will be allowed to run or not.
In addition to already available built-in policies, this enables high levels of customization, the only limit being the type of data that can be extracted that way.
The script has to be written using IF conditions, giving back only two outcomes:
Exit code 1: script execution result will be considered as "False"
Exit code 0: script execution result is considered to be “True”
In the example below, we are going to set a script that will allow the end users to run TDA only Monday to Friday, between 9 and 5 PM.
1. Open your profile and click on "Access Policies Rules" right click and then-→ “Add”:
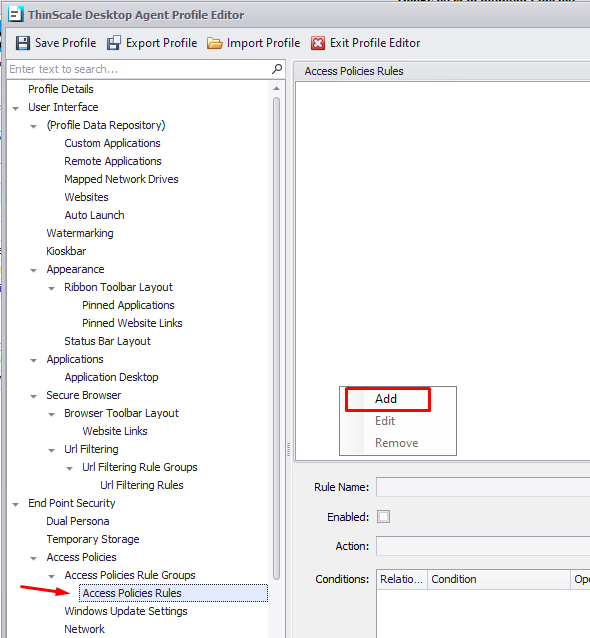
2. New Rule window will open. Add the desired name and select “Script Execution Result” from the dropdown. Once selected, click on the cogs symbol, which will bring you to the script customization screen:
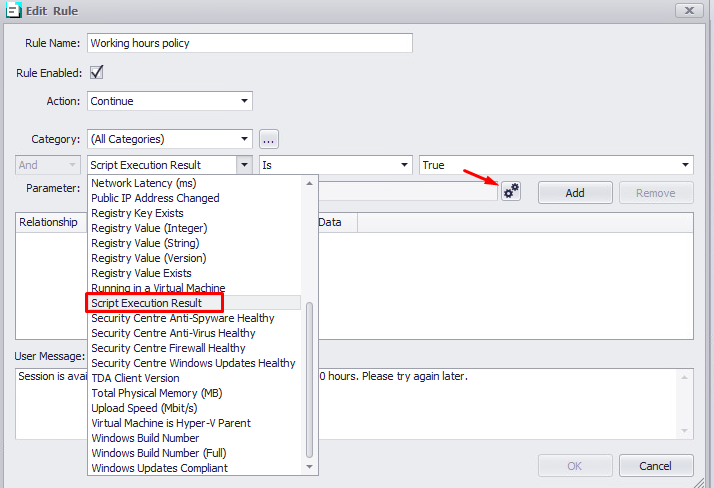
3. Input your script here, please ensure .ps1 is selected (script examples can be found later in the article):
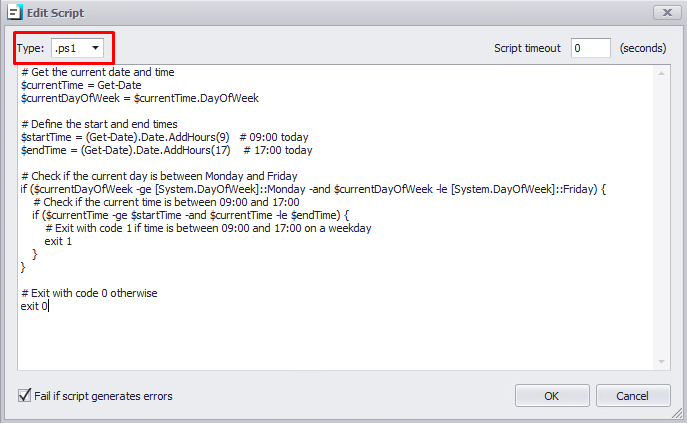
4. With the script in place, all that's left is to set the Action TDA will execute whether the script result comes back as True or False. As we want TDA to close if the user tries to run it outside the specified time period, we are going to set it to Close TDA if the result is True (Exit Code 0)
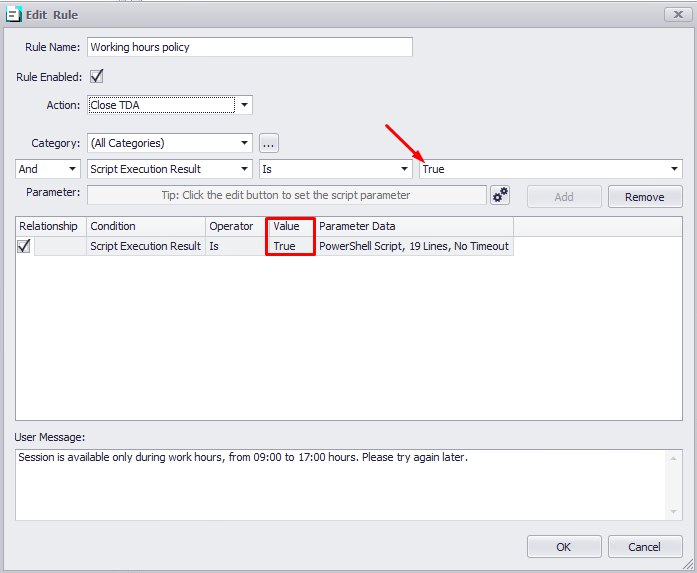
5. Additionally, we can add another script/rule to ensure time & date is set to automatic, to prevent users from changing it manually:
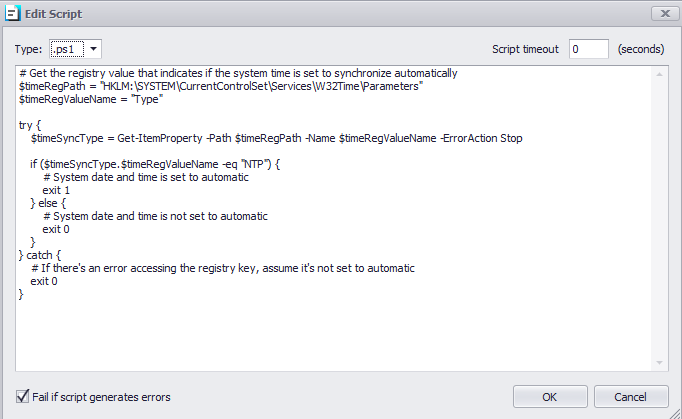
The final rule should look similar to the below:
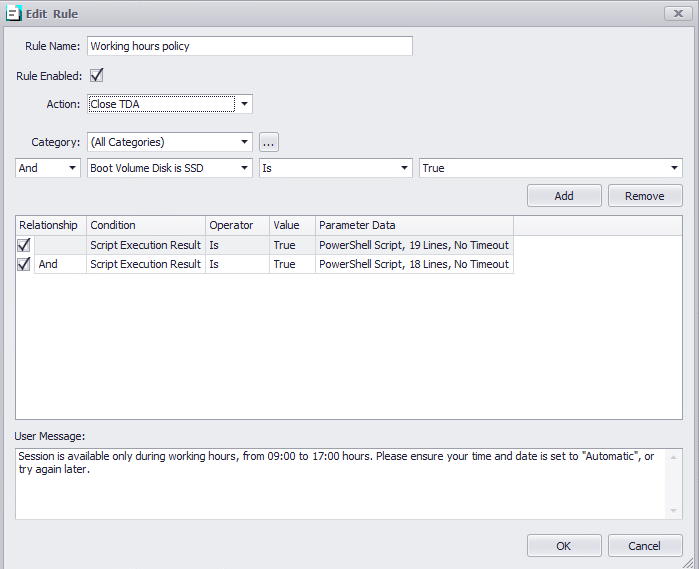
Example scripts collection:
Working hours policy
# Get the current date and time
$currentTime = Get-Date
$currentDayOfWeek = $currentTime.DayOfWeek
# Define the start and end times
$startTime = (Get-Date).Date.AddHours(9) # 09:00 today
$endTime = (Get-Date).Date.AddHours(17) # 17:00 today
# Check if the current day is between Monday and Friday
if ($currentDayOfWeek -ge [System.DayOfWeek]::Monday -and $currentDayOfWeek -le [System.DayOfWeek]::Friday) {
# Check if the current time is between 09:00 and 17:00
if ($currentTime -ge $startTime -and $currentTime -le $endTime) {
# Exit with code 1 if time is between 09:00 and 17:00 on a weekday
exit 1
}
}
# Exit with code 0 otherwise
exit 0
# Get the registry value that indicates if the system time is set to synchronize automatically
$timeRegPath = "HKLM:\SYSTEM\CurrentControlSet\Services\W32Time\Parameters"
$timeRegValueName = "Type"
try {
$timeSyncType = Get-ItemProperty -Path $timeRegPath -Name $timeRegValueName -ErrorAction Stop
if ($timeSyncType.$timeRegValueName -eq "NTP") {
# System date and time is set to automatic
exit 1
} else {
# System date and time is not set to automatic
exit 0
}
} catch {
# If there's an error accessing the registry key, assume it's not set to automatic
exit 0
}
Minimum screen resolution Full HD
# Get screen resolution
$screenResolution = (Get-CimInstance -Namespace root\wmi -ClassName WmiMonitorBasicDisplayParams).VideoOutputTechnology |
Select-Object -ExpandProperty VideoOutputTechnology |
ForEach-Object {
Get-WmiObject -Namespace root\wmi -ClassName WmiMonitorBasicDisplayParams |
Select-Object -Property ActiveWidth, ActiveHeight
} |
Sort-Object -Property ActiveWidth, ActiveHeight -Descending |
Select-Object -First 1
$width = $screenResolution.ActiveWidth
$height = $screenResolution.ActiveHeight
# Check if the resolution is 1920x1080 or higher
if ($width -ge 1920 -and $height -ge 1080) {
exit 1
} else {
exit 0
}
Active webcam check
# Get all devices with "webcam" or "camera" in their description (case-insensitive)
$webcamDevices = Get-PnpDevice -PresentOnly | Where-Object {
$_.Description -ilike "*webcam*" -or $_.Description -ilike "*camera*" -or
$_.FriendlyName -ilike "*webcam*" -or $_.FriendlyName -ilike "*camera*"
}
if ($webcamDevices) {
# Check if at least one webcam is enabled
$enabledWebcam = $webcamDevices | Where-Object { $_.Status -eq "OK" }
if ($enabledWebcam) {
Write-Output "Enabled webcam(s) found:" # Provide feedback to the user
$enabledWebcam | Format-Table FriendlyName, Status # Display a table with details
exit 0
} else {
Write-Output "Webcam(s) found, but all are disabled."
exit 1
}
} else {
Write-Output "No webcam detected."
exit 1
}
Laptop power check (battery or plugged-in)
$batteryStatus = (Get-CimInstance -ClassName Win32_Battery).BatteryStatus
if ($batteryStatus -eq 2) {
Write-Output "Laptop is plugged in."
exit 0 # Exit code 0 for plugged in
} else {
Write-Output "Laptop is on battery power."
exit 1 # Exit code 1 for on battery
}
Java version check
# Define the desired Java version to compare against (with wildcard)
$desiredJavaVersion = "1.8.0*"
# Function to compare Java versions
function Compare-JavaVersions {
param (
[string]$installedVersion,
[string]$desiredVersion
)
# Remove wildcard for comparison if it exists
if ($desiredVersion -like "*") {
$desiredVersion = $desiredVersion.TrimEnd('*')
}
# Split versions into components
$installedVersionComponents = $installedVersion -replace '_', '.' -split '\.'
$desiredVersionComponents = $desiredVersion -split '\.'
# Compare each component of the versions
for ($i = 0; $i -lt $desiredVersionComponents.Length; $i++) {
if ($installedVersionComponents[$i] -gt $desiredVersionComponents[$i]) {
Write-Output "Installed Java version ($installedVersion) is newer than the desired version ($desiredVersion)."
exit 0
} elseif ($installedVersionComponents[$i] -lt $desiredVersionComponents[$i]) {
Write-Output "Installed Java version ($installedVersion) is older than the desired version ($desiredVersion)."
exit 1
}
}
Write-Output "Installed Java version ($installedVersion) matches the desired version ($desiredVersion)."
exit 0
}
# Execute the java -version command and capture the output
$javaVersionOutput = & java -version 2>&1
# Check if the command executed successfully
if ($javaVersionOutput) {
# Parse the output to extract the version information
$javaVersionLine = $javaVersionOutput | Select-String -Pattern 'version'
if ($javaVersionLine) {
$javaVersion = $javaVersionLine -replace 'java version "|"', '' -replace 'openjdk version "|"', ''
$javaVersion = $javaVersion.Split(" ")[0].Trim()
Write-Output "Installed Java version: $javaVersion"
# Compare installed Java version with the desired version
Compare-JavaVersions -installedVersion $javaVersion -desiredVersion $desiredJavaVersion
} else {
Write-Output "Java version information not found."
exit 1
}
} else {
Write-Output "Java is not installed or the command failed to execute."
exit 1
}
VPN connection active check
# Get all network adapters with their status using CIM
$networkAdapters = Get-CimInstance -ClassName Win32_NetworkAdapter | Where-Object { $_.NetConnectionStatus -eq 2 }
# Filter to only adapters that are VPNs
$vpnAdapters = $networkAdapters | Where-Object { $_.NetConnectionID -like "*VPN*" -or $_.Name -like "*VPN*" }
# Check if there are any VPN adapters active
if ($vpnAdapters) {
Write-Host "Exiting with code 0 (VPN is active)"
exit 0 # VPN is active, exit with code 0
} else {
Write-Host "Exiting with code 1 (No VPN active)"
exit 1 # No VPN is active, exit with code 1
}